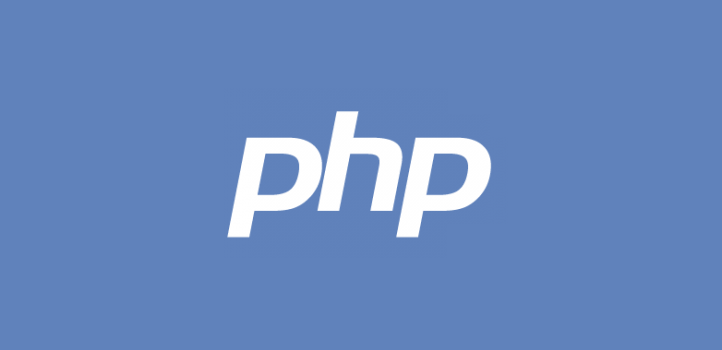
isset — Determine if a variable is declared and is different than NULL
Let’s try to print a undefined variable.
<?php
echo $name;
// will get error
?>
We get the error but we don’t want any error. We want build our application bug free and for that we need to kick all the possible error one by one.
isset
can help me in kicking lots of possible error. Let’s see how isset
will help us in avoiding the possible bugs.
Example 1 #
If there is any possibilities that the variable could be NULL or undeclared then it’s better to check it before using.
First let’s see how it works then I’ll tell you some use cases which you should always keep in your mind. These all use cases are based on my experience.
<?php
if(isset($name)){
echo $name;
}else{
echo "Name is not defined";
}
?>
Example 2 #
Whenever any user submit/send the form/data to the server. There is possibilities that all the fields are not sent by the user.
There is article about this only. You can read it to handle your form in more better way and make you form submission bug free.
<?php
if(isset($_POST)){
// This page is being requested using `POST method`.
if(isset($_POST['name'] , $_POST['email'] , $_POST['password'])){
// User sent `name` `email` and `password`.
}else{
// User missed either `name`, `email`, `password` or all the data.
}
}else{
// This page is not being called using `POST method`.
}
?>